ASP.NET Web server controls are ASP.NET specific objects or tags understood by the ASP.NET engine running in IIS.
Like HTML server controls, web server controls are also processed server-side by the ASP.NET engine so these controls require a runat="server"
attribute to work properly. Unlike HTML Server controls, Web server controls do not necessarily map to specific HTML elements.
In some cases, a Web server control may result in multiple HTML elements. For example, a TextBox
control might render as an input
tag or a textarea
tag, depending on its properties.
Web Server Control Syntax
The syntax for creating a Web server control is as follows:
<asp:controlName id="controlId" runat="server" />
ASP.NET provides these Web server controls to help you with rapid development. Unlike traditional HTML elements, server controls allow you to access different properties using server-side scripting.
When using Web server controls, you need to ensure that you include the runat="server"
attribute. This will allow you to access the control’s properties within your code blocks, for example during a page load, or click event.
Web Server Controls
Web server controls include traditional form controls such as buttons and text boxes as well as other controls such as tables. They also include controls that provide commonly used form functionality such as displaying data in a grid, displaying menus, and so on.
Here is a listing of some of the common Web Server Controls included in ASP.NET.
Starndard Web Server Controls
Control | Description |
---|---|
AdRotator Web Server Control | Provides a convenient way to display advertisements on your ASP.NET Web pages |
BulletedList Web Server Control | Creates an unordered or ordered list of items, which renders as an HTML ul or ol element |
Button Web Server Controls | Allow users to send a command via button, linkbutton, or imagebutton |
Calendar Web Server Control | Displays a one-month calendar |
CheckBox and CheckBoxList Controls | Provide a way for users to switch between true-false for single and group boxes |
DropDownList Web Server Control | Enables users to select from a single-selection drop-down list box |
FileUpload Web Server Control | Enables you to provide users with a way to send a file from their computer to the server |
HiddenField Web Server Control | Enables you to keep information in an ASP.NET Web page without displaying it to users |
HyperLink Web Server Control | Provides a means of creating and manipulating links on a Web page from server code |
Image Web Server Control | Enable you to display images on a Web Forms page |
ImageMap Web Server Control | Enables you to create an image that has individual regions that users can click |
Label Web Server Control | Provides a way to display text programmatically;rendered as a span element |
ListBox Web Server Control | Allows users to select one or more items from a predefined list |
Literal Web Server Control | Renders static text into a Web page without adding any HTML element |
Localize Web Server Control | Enables you to display localized text in a specific area |
MultiView and View Controls | Act as containers for other controls and markup, and present alternate views of information |
Panel Web Server Control | Provides a container within the page for other controls |
PlaceHolder Web Server Control | Enables you to place an empty container control, then dynamically add child elements at run time |
RadioButton & RadioButtonList Controls | Allow users to select one item or from a predefined list |
Substitution Web Server Control | Specifies a section on an output-cached Web page that is exempt from caching |
Table, TableRow, & TableCell Controls | Creates a general-purpose table |
TextBox Web Server Control | Provides a way for users to type information into an ASP.NET Web page |
Wizard Web Server Control | Enables you to build ASP.NET Web pages that present users with multi-step procedures |
XML Web Server Control | Reads XML and writes it into an ASP.NET Web page at the location of the control |
Data Server Controls
Control | Description |
---|---|
GridView Web Server Control | Displays data as a table. Provides sorting, paging, editing, or deleting records |
DetailsView Web Server Control | Renders a single record at a time as a table. Allows for paging and editing records |
FormView Web Server Control | Renders a single record at a time from a data source and provides paging of records |
Repeater Web Server Control | Renders a read-only list from a set of records returned from a data source |
DataList Web Server Control | Renders data as table and enables you to display data records in different layouts |
SqlDataSource Web Server Control | Enables you to work with Microsoft SQL Server, OLE DB, ODBC, or Oracle databases |
AccessDataSource Web Server Control | Enables you to work with a Microsoft Access database |
ObjectDataSource Web Server Control | Enables you to work with a business object and create applications that rely on middle-tier objects |
XmlDataSource Web Server Control | Enables you to work with an XML file |
SiteMapDataSource Web Server Control | Used with ASP.NET site navigation |
Validation Server Controls
Validator | Description |
---|---|
RequiredFieldValidator | Ensures that the user does not skip an entry |
CompareValidator | Compares a user’s entry against a constant value, against the value of another control, or for a specific data type |
RangeValidator | Checks that a user’s entry is between specified lower and upper boundaries |
RegularExpressionValidator | Checks that the entry matches a pattern defined by a regular expression |
CustomValidator | Checks the user’s entry using validation logic that you write yourself |
Navigation Server Controls
Control | Description |
---|---|
Menu Web Server Control | Enables you to add functionality to your web pages that are often used to provide navigation |
SiteMapPath Web Server Control | Displays a navigation path, also known as a breadcrumb |
Treeview Web Server Control | Displays hierarchical data, such as a table of contents or file directory, in a tree structure |
Login Server Controls
Control | Description |
---|---|
Login Control | Displays a user interface for user authentication |
LoginView Control | Allows you to display different information to anonymous and logged-in users |
LoginStatus Control | Displays a login link for users who are not authenticated and a logout link for users who are authenticated |
LoginName Control | Displays a user’s login name if the user has logged in using ASP.NET membership |
PasswordRecovery Control | Allows user passwords to be retrieved based on the e-mail address that was used when the account was created |
Web Parts Server Controls
Control | Description |
---|---|
AppearanceEditorPart Control | Provides an editor control that enables users to edit several UI properties on an associated WebPart control |
BehaviorEditorPart Control | Provides an editor control that enables users to edit properties that affect the behavior of an associated WebPart |
CatalogZone Control | Serves as the primary control in the Web Parts control set for hosting CatalogPart controls |
ConnectionsZone Control | Provides a UI that enables users to form connections between WebPart and other server controls |
DeclarativeCatalogPart Control | Enables you to add a catalog of WebPart or other server controls to a Web page |
EditorZone Control | Serves as the primary control in the Web Parts control set for hosting EditorPart controls |
ImportCatalogPart Control | Imports a description file for a WebPart control |
LayoutEditorPart Control | Provides an editor control that enables users to edit several layout-oriented UI properties |
PageCatalogPart Control | Provides a catalog that keeps references to all WebPart controls that a user has closed |
PropertyGridEditorPart Control | Provides an editor control that enables end users to edit custom properties on an associated WebPart |
ProxyWebPartManager Control | Provides a way to declare static connections in a content page |
WebPartZone Control | Serves as the primary control in the Web Parts control set for hosting WebPart controls |
Example
In the following example, the markup is located in the .aspx
file, and the VB or C# code is either in a script block within the .aspx
page or in the code-behind page. The .aspx
file contains a Hyperlink Standard Web Server Control.
ASPX
<asp:HyperLink ID="HyperLink1" runat="server" />
VB
Sub Page_Load(sender As Object, e As EventArgs)
HyperLink1.NavigateUrl = "https://www.itgeared.com"
End Sub
C#
void Page_Load(object sender, EventArgs e)
{
HyperLink1.NavigateUrl = "https://www.itgeared.com";
}
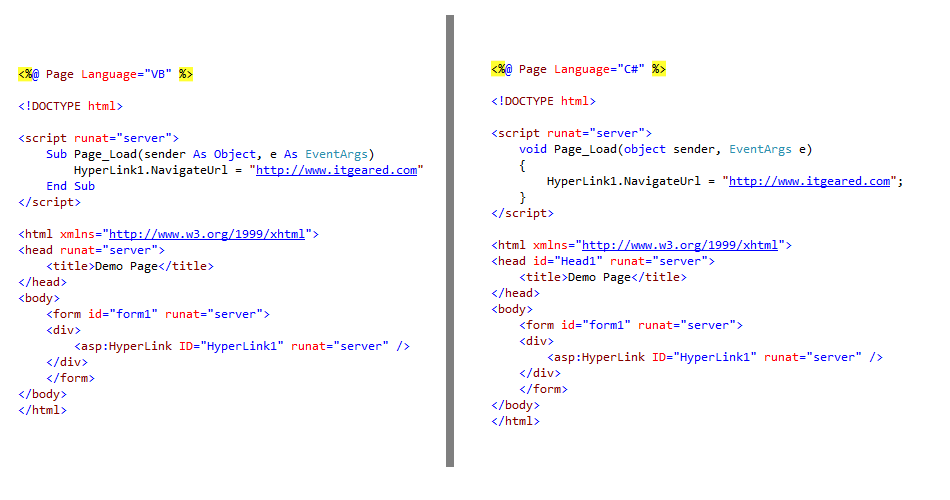
Another great resource to access is the MSDN Library found on Microsoft’s website.